Let’s put a function on the projectile.
I wanted to add a penetrate feature, but I didn’t know how to do it. So I tried using chatgpt.
First, I wrote this on chatgpt
Make me a projectile that goes through with Unity Playmaker
Below is the answer to that
Creating a “penetrating projection” in Unity using Playmaker, which is a visual scripting tool for Unity, involves a few steps. This example will show you how to set up a simple projectile that moves forward, detects collisions, and interacts with objects in Unity.
Here’s a step-by-step guide to creating a basic projectile in Unity using Playmaker:
Step 1: Setting Up the Unity Scene
- Create a new Unity project or open an existing one.
- Create a new GameObject for the projectile:
- Go to
GameObject -> 3D Object -> Sphere
(or another shape you prefer for your projectile). - Rename it to “Projectile”.
- Go to
- Add a Rigidbody to the Projectile:
- Select the “Projectile” GameObject.
- In the Inspector, click
Add Component
and search forRigidbody
. - Set the
Mass
to a low value like0.1
.
Step 2: Installing Playmaker
- Import Playmaker into your project if you haven’t already:
- Go to
Window -> Asset Store
. - Search for “Playmaker” and import it into your project.
- Go to
- Open Playmaker Editor:
- Go to
Playmaker -> Playmaker Editor
.
- Go to
Step 3: Setting Up the Playmaker FSM for the Projectile
- Create an FSM (Finite State Machine) on the Projectile:
- Select the “Projectile” GameObject.
- In the Playmaker Editor, click
Add FSM
. - Rename the FSM to “ProjectileLogic”.
- Add a Move Forward Action:
- In the Playmaker Editor, select the State named “State 1”.
- In the
Action Browser
, search forTranslate
. - Add the
Translate
action to the state. - Set
Vector
to(0, 0, 1)
(assuming the projectile should move forward on the Z-axis). - Set
Space
toSelf
.
- Add a Collision Detection:
- Add a new state to handle collisions.
- In the
Events
tab, create a new event named “COLLISION”. - Add the
COLLISION
event to the state transitions. - In the first state (“State 1”), add a new action called
Collision Event
. - Set
Collision Enter
to the event “COLLISION”. - Add a transition to the new state on the “COLLISION” event.
- Handle Collision:
- In the collision handling state, add actions to handle what happens when the projectile hits something. For example:
Destroy Self
: To destroy the projectile upon collision.Send Event
: To trigger events or effects on the object it collides with.
- In the collision handling state, add actions to handle what happens when the projectile hits something. For example:
Step 4: Testing the Projectile
- Create a simple launcher to instantiate the projectile:
- Create an empty GameObject and name it “Launcher”.
- Write a simple script to instantiate the projectile when a key is pressed.
csharp using UnityEngine;
public class Launcher : MonoBehaviour
{
public GameObject projectilePrefab;
void Update()
{
if (Input.GetKeyDown(KeyCode.Space))
{
Instantiate(projectilePrefab, transform.position, transform.rotation);
}
}
}
- Attach the Launcher Script:
- Attach the script to the “Launcher” GameObject.
- Assign the “Projectile” prefab to the
projectilePrefab
field in the Inspector.
Step 5: Final Adjustments
- Set up the projectile prefab:
- Drag the “Projectile” GameObject into the Project window to create a prefab.
- Use this prefab in the launcher script.
- Test the scene:
- Play the scene and press the spacebar to launch the projectile.
- Observe the projectile moving forward and interacting with objects upon collision.
This setup provides a basic framework for a projectile that moves forward and detects collisions using Playmaker in Unity. You can further customize the behavior by adding more actions and states to the Playmaker FSM.
=> I think this part is important (Add a transition to the new state on the “COLLISION” event.)
Allowing the projectile to continue to move after a trigger event occurs rather than directly destroy.
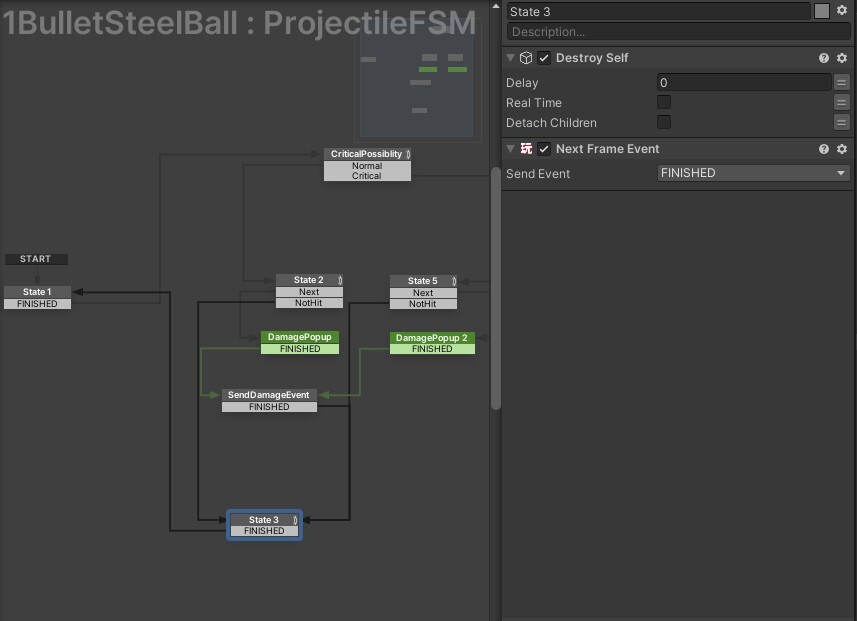
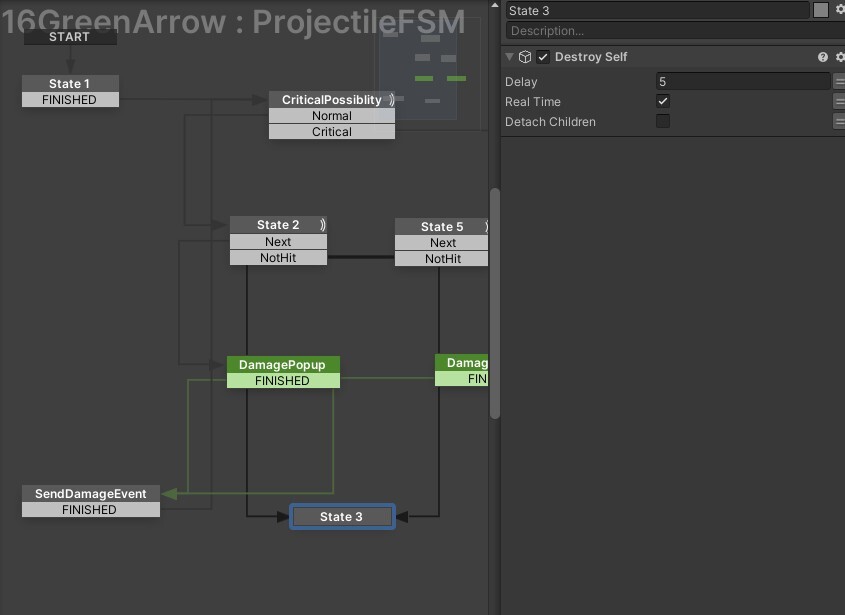
There was a five-second delay from the previous deletion.
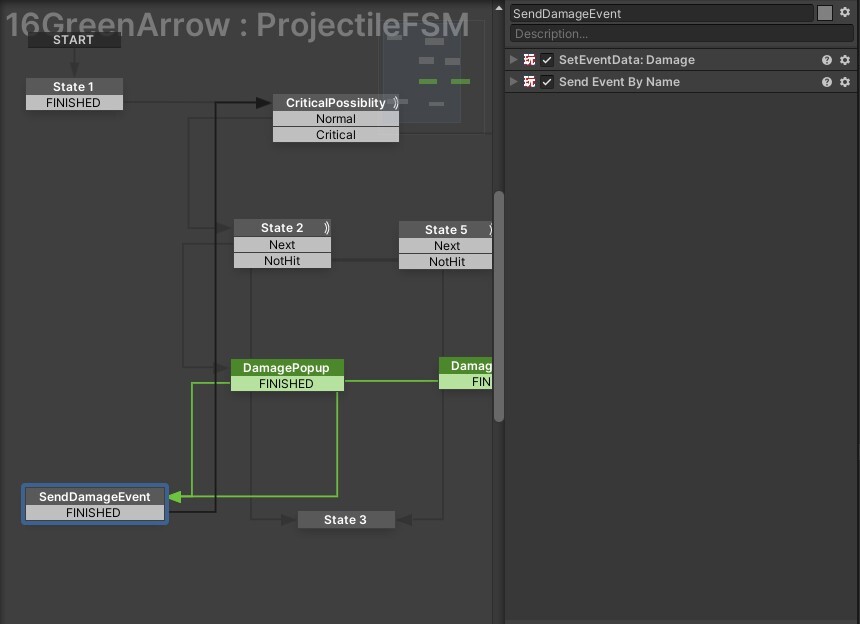
It allowed me to keep moving after triggering
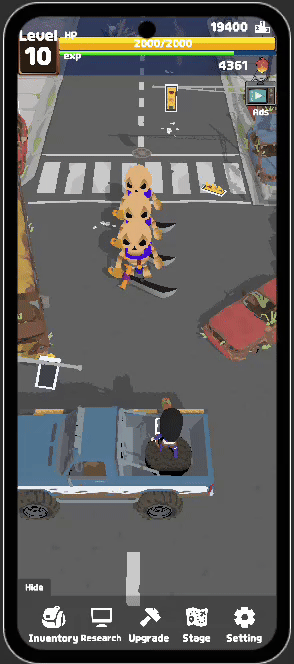
normal shot
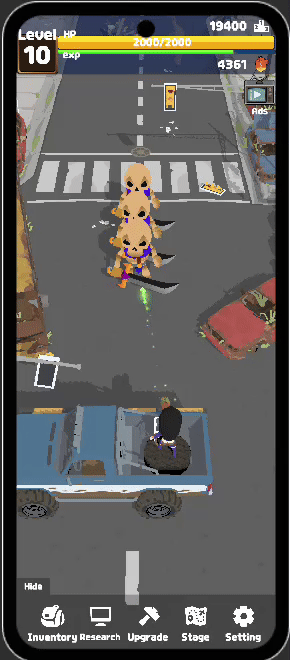
Penetrate Shot
I made the above result.